#TIL 32 - List all express routes
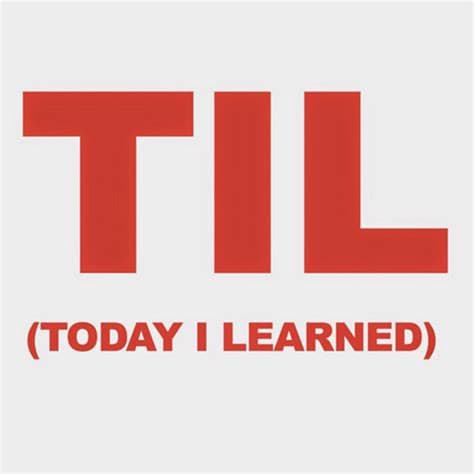
I'm pretty surprised this has been an open issue for ExpressJs for a long time.
- Create a helper for ExpressJs
/_ eslint-disable no-underscore-dangle _/
import { Application } from 'express';
// borrow from https://github.com/expressjs/express/issues/3308#issuecomment-618993790
function split(thing: any): string {
if (typeof thing === 'string') {
return thing;
}
if (thing.fast_slash) {
return '';
}
const match = thing
.toString()
.replace('\\/?', '')
.replace('(?=\\/|$)', '$')
.match(/^\/\^((?:\\[._+?^${}()|[\]\\/]|[^._+?^${}()|[\]\\/])*)\$\//);
return match ? match[1].replace(/\\(.)/g, '$1') : `<complex:${thing.toString()}>`;
}
function getRoutesOfLayer(path: string, layer: any): string[] {
if (layer.method) {
return [`${layer.method.toUpperCase()} ${path}`];
}
if (layer.route) {
return getRoutesOfLayer(path + split(layer.route.path), layer.route.stack[0]);
}
if (layer.name === 'router' && layer.handle.stack) {
let routes: string[] = [];
layer.handle.stack.forEach((stackItem: any) => {
routes = routes.concat(getRoutesOfLayer(path + split(layer.regexp), stackItem));
});
return routes;
}
return [];
}
export function getRoutes(app: Application): string[] {
let routes: string[] = [];
app.\_router.stack.forEach((layer: any) => {
routes = routes.concat(getRoutesOfLayer('', layer));
});
return routes;
}
export default { getRoutes };
- Then call from your main app
import { getRoutes } from './express/helper';
...
// show load routes on dev mode
try {
const allRoutes = getRoutes(app);
logger.info('all routes', allRoutes);
} catch (error) {
logger.error(error);
}